Chapter 3 Functions
As mentioned in previous chapter, R packages bundles functions so that are ready and easy available for users. That tells us that the core ingredients of R packages are functions. R packages contains several functions that together make a packages that is aimed to facilitate data import, manipulation and plotting within R environment (R Core Team 2020). Therefore, in this chapter we are going to learn how to make a simple function that work. We are not going to load with you with creating many functions, but we understand that once you master the basic of creating your own function, it will be easy to make more function that are intended to solve a particular data science probem.
We create a function and assign it a name max_min
. This function aim to find the difference of numeric values in a vector.
Let’s check whether the function work using the famous iris dataset. We only check the difference of variable Sepal.Length
## [1] 3.6
That work, what if we use a Species
variable
We get an error, but the message isn’t descriptive. That is common. For us to make descriptive notification when a wrong type of data is provided, we need to add the if-stop
function to validity the arguments that are required in the function. We recreate the function and additional arguments to check the validity of the arguments.
max_min <- function(x){
if(!is.numeric(x)){
stop("i am sorry, I can work with a ", class(x)[1], " variable you provided, Please supply me with numeric vector variable")
}
max(x) - min(x)
}
Let’s check again the variables species in our functions.
Woolah! now we get a descriptive error notification, what if we supply the numeric variable to the function!
## [1] 2.4
Let us add our first function max_min
to babye package using usethis::use_r("max_min"). This creates a black R script named
max_min.Rlocated in the
R/` sub-folder in our package directory. It is recommended that the R script and the function bear the same name. Then fill the R script of R function we created with the argument as shown in figure 3.1.
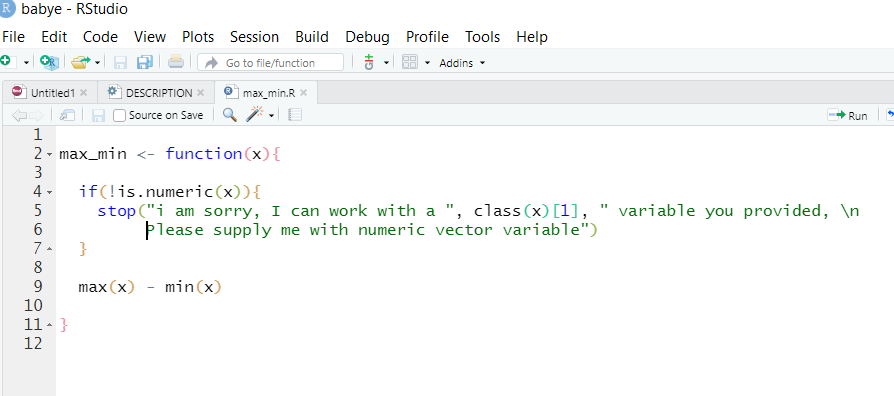
Figure 3.1: Function script
Once you have created the function, take your function for a test drive with devtools::load_all (“arguably the most important part of the devtools workflow”)
. devtools::load_all()
places your function in local memory so that you may tinker and confirm its execution. Let’s give it a try.
3.1 Document
Next document the max_min
function using the Roxygen skeleton. The roxygen2 package provides an in-source documentation system that automatically generates R documentation (Rd) files. First, place your cursor in the function definition, and then you can add the skeleton from code > insert Roxygen skeleton
in the Rstudio main tab (see figure 3.2).
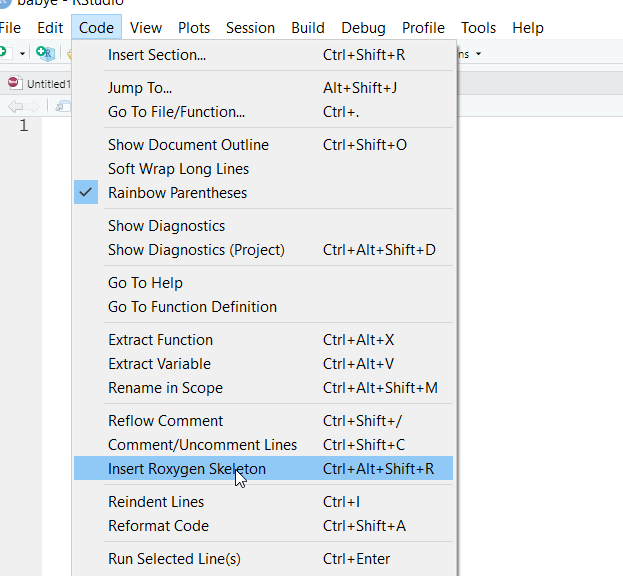
Figure 3.2: Inserting function documentation in Rstuido
You will get skeleton of documentation for the function, you can fill the field and updates it as you wish. This document the function and briefly describe it, which make it easy for you and user later that help instruct how to use the function. I have updated the max_min
function as seen in figure 3.3
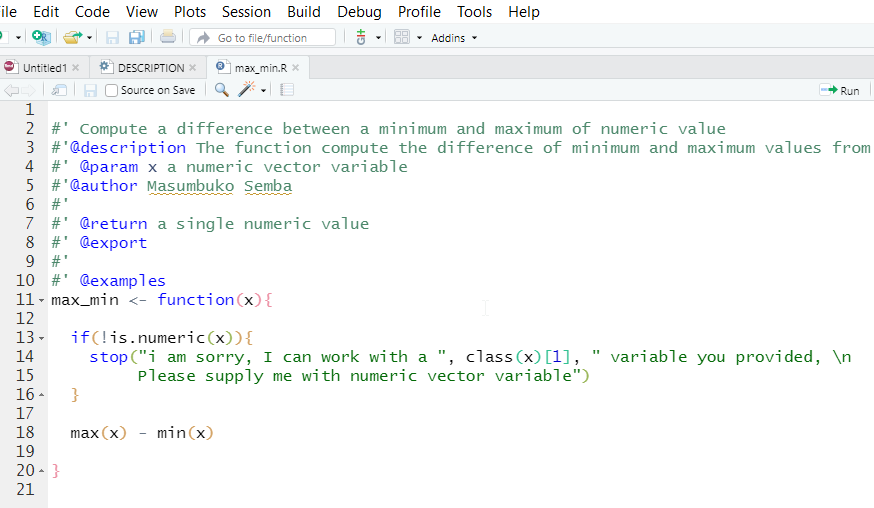
Figure 3.3: documented function
Once you have update the function document, save it and submit using the devtools::document
function.
3.2 Dependencies
It’s the job of the DESCRIPTION to list the packages that your package needs to work. To specify a package dependency, the name of the package needs to be listed in the DESCRIPTION file. This can be automatically done for you by submitting.

Figure 3.4: Package dependency
We notice that our package to work with pipe operator, it requires a magritrr package (???). That means when you install this package, it will also install the packages it depends like magritrr.
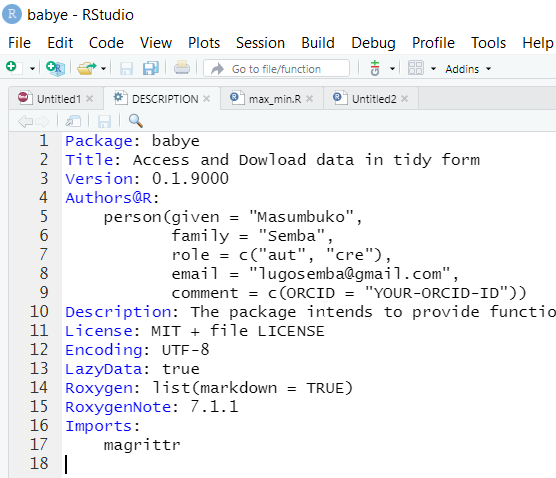
Figure 3.5: Package dependency
3.3 Special Case
Although we have imported a magritrr package, this package has special operator called pipe or then denoted as %>%
, which provide a convenient way to chain the processes in R. We can add this special function in two steps
usethis::use_pipe()
—which createsutils-pipe.R
folder and addsmagritrr
to imports in the DESCRIPTION file.devtools::document()
—add the pipe in our package NAMESPACE file.
References
R Core Team. 2020. R: A Language and Environment for Statistical Computing. Vienna, Austria: R Foundation for Statistical Computing. https://www.R-project.org/.